Zolitude V1.0
Zolitude » Devlog
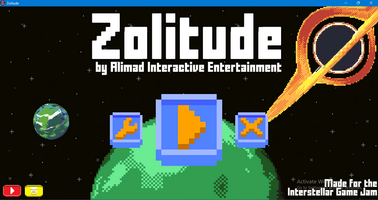
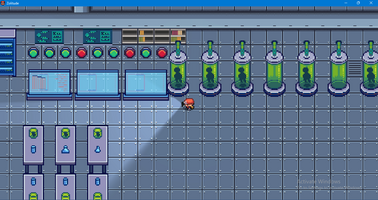
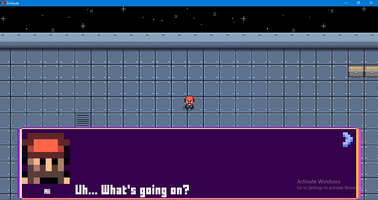
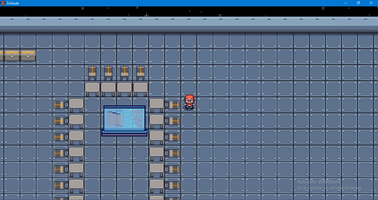
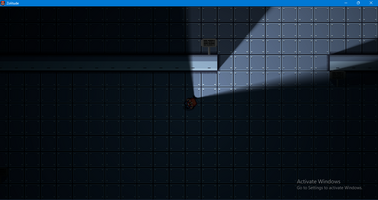
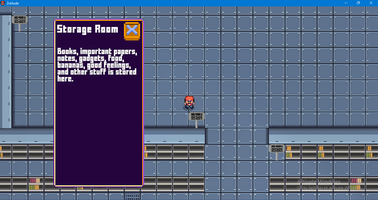
Alright, there are a few solutions to some problems that I found while making this game. And I would like to share some of them.
Advanced Dialogue System & Tilemap Optimization
I used an advanced dialogue system to handle the dialogue and not to write everything in the code. I found it very convenient with callbacks.
///////////////////////////////////////////////// //////////////The DialogueManager/////////////// ///////////////////////////////////////////////// public class Canva : MonoBehaviour { public Sprite[] chars; public Sprite[] charsopenmouth; public string curr; public Image face; public TextMeshProUGUI dialog; public GameObject box; public string[] dialogs; public int[] dialogchars; public int[] callbacks; public int currentdialogue; public bool speaking, mouthopen; public string nextDial; public int nChar; public AudioClip open, close; public AudioSource src; public TextMeshProUGUI name; void Start() { InvokeRepeating("myUpdate", 0, 0.15f); InvokeRepeating("mySecUpdate", 0, 0.04f); beginDelay(1, 2f); Global.dialogueManager = this; name.text = Global.name; } private void mySecUpdate() { if (speaking) { if (curr != nextDial) { curr += nextDial[nChar]; nChar++; } else { speaking = false; } dialog.text = curr; } } void myUpdate() { src.volume = speaking ? 0.8f : 0.0f; if (speaking) { mouthopen = !mouthopen; } else { } face.sprite = mouthopen ? chars[dialogchars[currentdialogue]] : charsopenmouth[dialogchars[currentdialogue]]; } public void next() { AudioSource.PlayClipAtPoint(close, Global.player.transform.position); if (!speaking) { if (callbacks[currentdialogue] == 0) { if (currentdialogue == 5) { GameObject.FindGameObjectWithTag("Player").GetComponent<Player>().viewCtrls(); } if (currentdialogue == 28) { GameObject.FindGameObjectWithTag("Player").GetComponent<Player>().StartCoroutine(GameObject.FindGameObjectWithTag("Player").GetComponent<Player>().end()); } curr = ""; dialog.text = ""; box.SetActive(false); } else { currentdialogue = callbacks[currentdialogue]; speaking = true; box.SetActive(true); speaking = true; nextDial = dialogs[currentdialogue]; curr = ""; dialog.text = ""; nChar = 0; } } else { curr = nextDial; } } public void beginDelay(int dial, float delay) { StartCoroutine(bD(dial, delay)); } public IEnumerator bD(int dial, float delay) { float d = delay; while (delay > 0) { delay -= Time.deltaTime; yield return null; } begin(dial); } public void begin(int dial) { AudioSource.PlayClipAtPoint(open, Global.player.transform.position); box.SetActive(true); currentdialogue = dial; speaking = true; nextDial = dialogs[currentdialogue]; curr = ""; nChar = 0; myUpdate(); } } ///////////////////////////////////////////////// //////////////The Tilemap Renderer/////////////// ///////////////////////////////////////////////// public class OptimizedTilemapRenderer : MonoBehaviour { public int visibleWidth = 20; // Number of tiles visible horizontally public int visibleHeight = 15; // Number of tiles visible vertically private Tilemap tilemap; private Camera mainCamera; private Vector3Int previousCameraTilePosition; void Start() { tilemap = GetComponent<tilemap>(); getCam(); InvokeRepeating("getCam", 0f, 5f); previousCameraTilePosition = new Vector3Int(int.MinValue, int.MinValue, int.MinValue); MakeAllTilesInvisible(); UpdateVisibleTiles(); } void MakeAllTilesInvisible() { BoundsInt bounds = tilemap.cellBounds; foreach (Vector3Int pos in bounds.allPositionsWithin) { TileBase tile = tilemap.GetTile(pos); if (tile != null) { tilemap.SetTileFlags(pos, TileFlags.None); tilemap.SetColor(pos, Color.clear); } } } public void getCam() { foreach (GameObject camera in GameObject.FindGameObjectsWithTag("PlayerCam")) { if (camera.activeSelf) { mainCamera = camera.GetComponent<camera>(); break; } } } void Update() { UpdateVisibleTiles(); } void UpdateVisibleTiles() { if (mainCamera == null) return; Vector3 cameraPosition = mainCamera.transform.position; Vector3Int cameraTilePosition = tilemap.WorldToCell(cameraPosition); if (cameraTilePosition != previousCameraTilePosition) { int halfWidth = visibleWidth / 2; int halfHeight = visibleHeight / 2; Vector3Int min = new Vector3Int(cameraTilePosition.x - halfWidth, cameraTilePosition.y - halfHeight, 0); Vector3Int max = new Vector3Int(cameraTilePosition.x + halfWidth, cameraTilePosition.y + halfHeight, 0); // Hide tiles that are no longer in view for (int x = previousCameraTilePosition.x - halfWidth; x <= previousCameraTilePosition.x + halfWidth; x++) { for (int y = previousCameraTilePosition.y - halfHeight; y <= previousCameraTilePosition.y + halfHeight; y++) { if (x < min.x || x > max.x || y < min.y || y > max.y) { Vector3Int pos = new Vector3Int(x, y, 0); TileBase tile = tilemap.GetTile(pos); if (tile != null) { tilemap.SetTileFlags(pos, TileFlags.None); tilemap.SetColor(pos, Color.clear); } } } } // Show tiles that are now in view for (int x = min.x; x <= max.x; x++) { for (int y = min.y; y <= max.y; y++) { if (x < previousCameraTilePosition.x - halfWidth || x > previousCameraTilePosition.x + halfWidth || y < previousCameraTilePosition.y - halfHeight || y > previousCameraTilePosition.y + halfHeight) { Vector3Int pos = new Vector3Int(x, y, 0); TileBase tile = tilemap.GetTile(pos); if (tile != null) { tilemap.SetTileFlags(pos, TileFlags.None); tilemap.SetColor(pos, Color.white); } } } } previousCameraTilePosition = cameraTilePosition; } } } </camera></tilemap>
Files
Zolitude Windows.zip 37 MB
Jun 10, 2024
Get Zolitude
Zolitude
2D Top Down Game
Status | Released |
Author | Alimad-co |
Genre | Adventure, Role Playing |
Tags | 2D, Horror, Singleplayer |
More posts
- Major Update to Zolitude!18 days ago
- Android UpdateJun 11, 2024
- Zolitude V1.0 UpdatedJun 10, 2024
Leave a comment
Log in with itch.io to leave a comment.